Git-ing Started (Low Hanging Fruit Sure Is Delicious)
Before getting started we should first learn one of the core pillars of software development of any kind — version control. We shall learn about one of the most ubiquitous names in the business, Git.
The first thing to know is the difference between Git, and names like GitHub, Bitbucket, GitLab etc. The way I prefer to think of it is that Git is the repository that lives in your computer, and places like GitHub (and Bitbucket, GitLab) are online repositories for your git repositories. A hub of gits, if you will.
So what we want is to set up a git repository for our local Unity project, and connect it to a remote GitHub repository. To begin, head over to git-scm.com and download the one for your OS.

Then, go ahead and run the setup executable. For our purposes it’s ok to go with the default option on everything except, in my opinion, the part about the name change option of the initial branch in repositories from “master” to something else, although “main” appears to be the new convention.

Once done, search “git bash” on your machine and click on it, it’ll open up the git console.

If you’re a Linux user then navigation should be no problem because it’s exactly the same. But fret not if this looks intimidating, just grasp some key concepts and you’re ready to go.
ls (list)

cd (change directory)
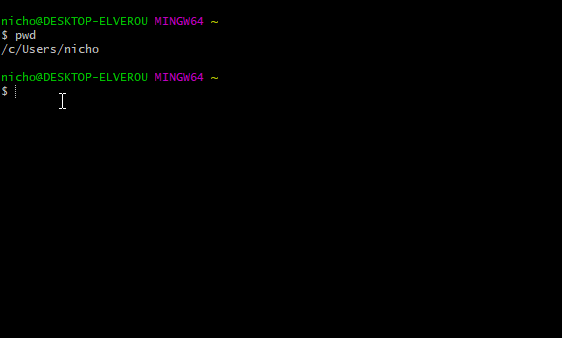
cd ..
cd Documents
cd “D:\Unity Projects”
cd can either be relative to where you currently are, so cd.. to go back a level like in the gif, or cd [folder] to go forward into a folder, or absolute file paths, such as cd “D:\Unity Projects” (double quotes help with passing in Windows filepaths as well as file names containing spaces)
GitHub repository set up
Let’s park git for now and head over to GitHub to set up an account and start a new repository

Pay attention to the option to add a .gitignore file and select Unity. This ensures unnecessary files aren’t pushed into GitHub, and there’s actually a lot of them you’ll see later! Then create the repository, and let’s return to the git stuff.
Navigate to your Unity project folder (a quick way is to go to the project directory in File Explorer, right click → Git Bash here). Then start by typing:
git init

This establishes a local git repository in your project. You now want to connect it to GitHub so head back to GitHub and copy the URL under the green Code button for the repository you made there earlier.

Back in git type:
git remote add origin [copied URL].
What this does it that it specifies your remote repository (called origin) and establishes the connection.

git remote -v
git remote -v verifies that the connection has been made.
Now there are three very important general git steps to keep in mind:
- pull (update your project from GitHub)
- commit (local snapshots of your progress)
- push (send that local progress to GitHub)
git pull origin main
So we start with pull. This pulls from the origin, into your main branch. Do this whenever you feel like you might want to update your project to have the latest possible version. For now it only gets the .gitignore file and adds it to your project folder.
git status
git status shows you what files haven’t been added for a commit later.

git add [file] OR git add . (to add all files/folders in there)
git add adds files to a sort of staging area of files to commit. The .gitignore file is very import for this step, otherwise you get the following case of trying to add files from the Library folder that aren’t actually needed:

git commit -m "Meaningful commit message here"
Once you confident you’re at a stage you want to take a snapshot of your progress, you git commit. You must add a message otherwise it will throw you into a VI text editor and then you’d need to lookup how to get out of that (hint it’s ESC + : + wq then press Enter)
git push origin main
Finally when you’re ready to share your progress to GitHub, perhaps for others to use, then you git push to origin the local things you’ve committed thus far.
And that’s the how to connect your Unity project’s git to GitHub! There’s also the Git Documentation that’s a great resource to learn all about the nuts and bolts, and you may want to google a git cheatsheet that best helps you understand for quick reference in future.